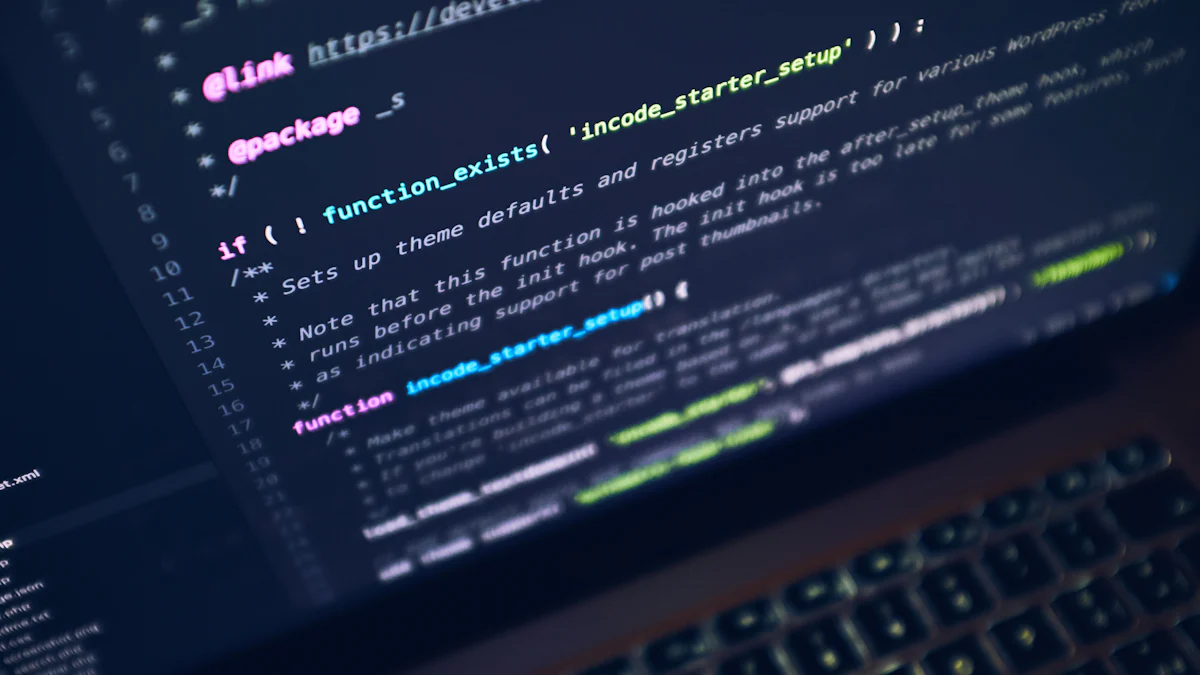
Python stands as a significant programming language in the tech world. Understanding important keywords in Python enhances coding efficiency and readability. Important keywords form the foundation of Python syntax. Mastering these important keywords allows developers to write clean and effective code. Important keywords also help in debugging and maintaining Python programs. Knowledge of important keywords is crucial for both beginners and experienced developers. Important keywords provide a structured approach to problem-solving in Python. Learning important keywords can lead to better job opportunities in the tech industry.
def
Definition
The def
keyword defines a function in Python. Functions encapsulate code blocks for reuse and
organization. The def
keyword signals the start of a function definition.
Syntax
The syntax for defining a function with the def
keyword follows:
def function_name(parameters):
# code block
return value
The function name identifies the function. Parameters within parentheses allow input values. The colon indicates the start of the function body. Indentation signifies the code block belonging to the function.
Examples
Example 1: A simple function that adds two numbers:
def add_numbers(a, b):
result = a + b
return result
Example 2: A function that prints a greeting message:
def greet(name):
print(f"Hello, {name}!")
Use Cases
Functions
Functions perform specific tasks within a program. Functions improve code readability and maintainability. Functions enable code reuse across different parts of a program.
Example: A function that calculates the factorial of a number:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
Methods
Methods are functions defined within a class. Methods operate on instances of the class. Methods provide behavior to objects created from the class.
Example: A method within a class representing a person:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
print(f"My name is {self.name} and I am {self.age} years old.")
The def
keyword serves as one of the important keywords in Python. Understanding the
def
keyword helps developers create efficient and organized code. Mastering the use of the
def
keyword proves essential for both beginners and experienced developers.
class
Definition
The class
keyword defines a new class in Python. Classes serve as blueprints for creating
objects. Each object created from a class can have attributes and methods.
Syntax
The syntax for defining a class with the class
keyword follows:
class ClassName:
# class attributes and methods
The class name identifies the class. Indentation signifies the code block belonging to the class.
Examples
Example 1: A simple class representing a car:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f"{self.year} {self.make} {self.model}")
Example 2: A class representing a bank account:
class BankAccount:
def __init__(self, owner, balance=0):
self.owner = owner
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if amount <= self.balance:
self.balance -= amount
else:
print("Insufficient funds")
Use Cases
Object-Oriented Programming
Object-Oriented Programming (OOP) relies on classes and objects. Classes encapsulate data and behavior. OOP promotes code reusability and modularity.
Example: Creating objects from the Car
class:
car1 = Car("Toyota", "Corolla", 2020)
car2 = Car("Honda", "Civic", 2019)
car1.display_info()
car2.display_info()
Inheritance
Inheritance allows a class to inherit attributes and methods from another class. The class
keyword supports inheritance by specifying a parent class.
Example: A class inheriting from another class:
class ElectricCar(Car):
def __init__(self, make, model, year, battery_size):
super().__init__(make, model, year)
self.battery_size = battery_size
def display_battery_info(self):
print(f"Battery size: {self.battery_size} kWh")
Creating an object from the ElectricCar
class:
electric_car = ElectricCar("Tesla", "Model S", 2021, 100)
electric_car.display_info()
electric_car.display_battery_info()
Understanding the class
keyword proves essential for mastering Python. The class
keyword stands among the important keywords in Python. Mastery of important keywords like class
enables developers to write robust and maintainable code.
if
Definition
The if
keyword creates conditional statements in Python. Conditional statements execute code
based on specific conditions. The if
keyword evaluates a condition and runs the code block if
the condition is true.
Syntax
The syntax for using the if
keyword follows:
if condition:
# code block
The condition represents an expression that evaluates to either true or false. Indentation signifies the code block that executes when the condition is true.
Examples
Example 1: A simple if
statement that checks if a number is positive:
number = 10
if number > 0:
print("The number is positive.")
Example 2: An if
statement that checks if a user is an adult:
age = 18
if age >= 18:
print("The user is an adult.")
Use Cases
Conditional Statements
Conditional statements control the flow of a program. The if
keyword allows decisions based on
conditions. Developers use conditional statements to handle different scenarios.
Example: Checking if a number is even or odd:
number = 4
if number % 2 == 0:
print("The number is even.")
else:
print("The number is odd.")
Control Flow
Control flow refers to the order in which code executes. The if
keyword directs the program's
control flow. Important keywords like if
help manage complex logic in programs.
Example: Determining the grade based on a score:
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
else:
print("Grade: F")
Understanding the if
keyword proves essential for mastering Python. The if
keyword
stands among the important keywords in Python. Mastery of important keywords like if
enables
developers to write robust and maintainable code.
else
Definition
The else
keyword provides an alternative code block that executes when an if
condition evaluates to false. The else
statement ensures that a specific block of code runs if
none of the preceding conditions are met.
Syntax
The syntax for using the else
keyword follows:
if condition:
# code block for true condition
else:
# code block for false condition
Indentation signifies the code blocks belonging to the if
and else
statements.
Examples
Example 1: An else
statement that handles a negative number:
number = -5
if number > 0:
print("The number is positive.")
else:
print("The number is not positive.")
Example 2: An else
statement that checks if a user is a minor:
age = 16
if age >= 18:
print("The user is an adult.")
else:
print("The user is a minor.")
Use Cases
Conditional Statements
Conditional statements control program flow based on conditions. The else
keyword provides a
fallback option. Developers use else
to handle scenarios where conditions do not meet.
Example: Checking if a number is zero or non-zero:
number = 0
if number != 0:
print("The number is non-zero.")
else:
print("The number is zero.")
Control Flow
Control flow refers to the order in which code executes. The else
keyword helps manage control
flow by providing alternative paths. Important keywords like else
simplify complex logic in
programs.
Example: Determining pass or fail based on a score:
score = 65
if score >= 70:
print("Pass")
else:
print("Fail")
Understanding the else
keyword proves essential for mastering Python. The else
keyword stands among the important keywords in Python. Mastery of important keywords like else
enables developers to write robust and maintainable code.
elif
Definition
The elif
keyword stands for "else if" in Python. Programmers use elif
to check
multiple conditions in a sequence. The elif
statement follows an if
statement and
precedes an else
statement.
Syntax
The syntax for using the elif
keyword follows:
if condition1:
# code block for condition1
elif condition2:
# code block for condition2
else:
# code block for all other conditions
Indentation signifies the code blocks belonging to each condition.
Examples
Example 1: An elif
statement that checks if a number is positive, negative, or zero:
number = 0
if number > 0:
print("The number is positive.")
elif number < 0:
print("The number is negative.")
else:
print("The number is zero.")
Example 2: An elif
statement that determines the size category of a person based on height:
height = 170
if height > 180:
print("Tall")
elif height > 160:
print("Average")
else:
print("Short")
Use Cases
Conditional Statements
Conditional statements control the flow of a program. The elif
keyword allows checking multiple
conditions in a structured manner. Developers use elif
to handle various scenarios efficiently.
Example: Determining the day type based on the day of the week:
day = "Wednesday"
if day == "Saturday" or day == "Sunday":
print("Weekend")
elif day == "Monday":
print("Start of the week")
elif day == "Friday":
print("End of the week")
else:
print("Midweek")
Control Flow
Control flow refers to the order in which code executes. The elif
keyword helps manage control
flow by providing multiple decision points. Important keywords like elif
simplify complex logic
in programs.
Example: Assigning a performance rating based on a score:
score = 75
if score >= 90:
print("Excellent")
elif score >= 75:
print("Good")
elif score >= 50:
print("Average")
else:
print("Poor")
Understanding the elif
keyword proves essential for mastering Python. The elif
keyword stands among the important keywords in Python. Mastery of important keywords like elif
enables developers to write robust and maintainable code.
for
Definition
The for
keyword creates loops in Python. Loops repeat a code block multiple times. The
for
keyword iterates over sequences like lists, tuples, and strings.
Syntax
The syntax for using the for
keyword follows:
for variable in sequence:
# code block
The variable represents each element in the sequence. Indentation signifies the code block that executes during each iteration.
Examples
Example 1: A for
loop that prints each item in a list:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Example 2: A for
loop that calculates the sum of numbers in a range:
total = 0
for number in range(1, 6):
total += number
print(total)
Use Cases
Loops
Loops perform repetitive tasks efficiently. The for
keyword simplifies iteration over sequences.
Important keywords like for
enhance code readability.
Example: A for
loop that prints each character in a string:
message = "Hello"
for char in message:
print(char)
Iteration
Iteration refers to the process of repeating steps. The for
keyword facilitates iteration
through sequences. Important keywords like for
streamline complex operations.
Example: A for
loop that squares each number in a list:
numbers = [1, 2, 3, 4]
squares = []
for number in numbers:
squares.append(number ** 2)
print(squares)
Understanding the for
keyword proves essential for mastering Python. The for
keyword stands among the important keywords in Python. Mastery of important keywords like for
enables developers to write efficient and maintainable code.
while
Definition
The while
keyword creates loops in Python. Loops repeat a code block as long as a condition
remains true. The while
keyword evaluates the condition before each iteration.
Syntax
The syntax for using the while
keyword follows:
while condition:
# code block
The condition represents an expression that evaluates to either true or false. Indentation signifies the code block that executes during each iteration.
Examples
Example 1: A while
loop that prints numbers from 1 to 5:
number = 1
while number <= 5:
print(number)
number += 1
Example 2: A while
loop that calculates the factorial of a number:
n = 5
factorial = 1
while n > 0:
factorial *= n
n -= 1
print(factorial)
Use Cases
Loops
Loops perform repetitive tasks efficiently. The while
keyword allows continuous execution based
on a condition. Important keywords like while
enhance code readability and functionality.
Example: A while
loop that prints even numbers less than 10:
number = 2
while number < 10:
print(number)
number += 2
Iteration
Iteration refers to the process of repeating steps. The while
keyword facilitates iteration
through conditions. Important keywords like while
streamline complex operations.
Example: A while
loop that sums numbers until the total exceeds 50:
total = 0
number = 1
while total <= 50:
total += number
number += 1
print(total)
Understanding the while
keyword proves essential for mastering Python. The while
keyword stands among the important keywords in Python. Mastery of important keywords like while
enables developers to write efficient and maintainable code.
try
Definition
The try
keyword initiates a block of code that tests for errors. Python uses try
to
handle exceptions gracefully. The try
block contains code that might raise an exception.
Syntax
The syntax for using the try
keyword follows:
try:
# code block to test
except ExceptionType:
# code block to handle the exception
Indentation signifies the code blocks belonging to the try
and except
statements.
Examples
Example 1: A try
block that handles division by zero:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
Example 2: A try
block that handles file not found error:
try:
file = open("nonexistent_file.txt", "r")
except FileNotFoundError:
print("File not found.")
Use Cases
Exception Handling
Exception handling manages errors without crashing the program. The try
keyword allows
developers to catch and handle exceptions. This approach ensures that the program continues running
smoothly.
Example: Handling multiple exceptions in a try
block:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ValueError:
print("Invalid input. Please enter a number.")
except ZeroDivisionError:
print("Cannot divide by zero.")
Error Management
Error management involves anticipating and resolving potential issues. The try
keyword helps
developers manage errors effectively. This practice improves the robustness of the code.
Example: Using a try
block to handle network errors:
import requests
try:
response = requests.get("https://example.com")
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except requests.exceptions.ConnectionError as conn_err:
print(f"Connection error occurred: {conn_err}")
Understanding the try
keyword proves essential for mastering Python. The try
keyword stands among the important keywords in Python. Mastery of important keywords like try
enables developers to write robust and maintainable code.
except
Definition
The except
keyword handles exceptions in Python. When an error occurs, the except
block catches and manages it. This prevents the program from crashing.
Syntax
The syntax for using the except
keyword follows:
try:
# code block that may raise an exception
except ExceptionType:
# code block to handle the exception
Indentation signifies the code blocks belonging to the try
and except
statements.
Examples
Example 1: An except
block that handles a division by zero error:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
Example 2: An except
block that handles a file not found error:
try:
file = open("nonexistent_file.txt", "r")
except FileNotFoundError:
print("File not found.")
Use Cases
Exception Handling
Exception handling ensures that programs run smoothly. The except
keyword allows developers to
catch and manage errors. This practice improves the robustness of the code.
Example: Handling multiple exceptions in a try
block:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ValueError:
print("Invalid input. Please enter a number.")
except ZeroDivisionError:
print("Cannot divide by zero.")
Error Management
Error management involves anticipating and resolving potential issues. The except
keyword helps
developers manage errors effectively. This approach enhances the reliability of the code.
Example: Using an except
block to handle network errors:
import requests
try:
response = requests.get("https://example.com")
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except requests.exceptions.ConnectionError as conn_err:
print(f"Connection error occurred: {conn_err}")
Understanding the except
keyword proves essential for mastering Python. The except
keyword stands among the important keywords in Python. Mastery of important keywords like
except
enables developers to write robust and maintainable code.
import
Definition
The import
keyword allows developers to include external modules in Python programs. Modules
contain reusable code that can be imported into different scripts. The import
keyword enhances
code organization and functionality.
Syntax
The syntax for using the import
keyword follows:
import module_name
Developers can also import specific functions or classes from a module:
from module_name import function_name
To avoid naming conflicts, developers can use aliases:
import module_name as alias
Examples
Example 1: Importing the math
module to perform mathematical operations:
import math
result = math.sqrt(16)
print(result)
Example 2: Importing the datetime
module to work with dates and times:
import datetime
current_date = datetime.date.today()
print(current_date)
Example 3: Importing a specific function from the random
module:
from random import randint
random_number = randint(1, 10)
print(random_number)
Use Cases
Module Importing
Module importing allows developers to leverage pre-written code. The import
keyword enables
access to a vast library of modules. Important keywords like import
facilitate the inclusion of
specialized functions and classes.
Example: Importing the os
module to interact with the operating system:
import os
current_directory = os.getcwd()
print(current_directory)
Code Reusability
Code reusability improves efficiency and reduces redundancy. The import
keyword promotes the
reuse of code across multiple projects. Important keywords like import
help maintain clean and
modular codebases.
Example: Importing a custom module to reuse functions:
# In file utilities.py
def greet(name):
return f"Hello, {name}!"
# In main script
import utilities
message = utilities.greet("Alice")
print(message)
Understanding the import
keyword proves essential for mastering Python. The import
keyword stands among the important keywords in Python. Mastery of important keywords like
import
enables developers to write efficient and maintainable code.
The ten most important keywords in Python include def
, class
, if
,
else
, elif
, for
, while
, try
,
except
, and import
. Mastering these important keywords enhances coding skills.
Practice and application of these important keywords in real-world projects improve proficiency. Continuous
learning and improvement in Python programming remain essential. Understanding important keywords leads to
better job opportunities and efficient problem-solving.